In this post we will see how to Upload and read a file in the apex controller through Lightning Component.
Though lightning has a file upload component here, sometimes we want to upload files to a third party system or do some other processing rather than attaching it to a Salesforce record.
To do that, we can use lightning file input component. The file contents can than be sent to Apex controller and processed further.
This is how a file input lightning component looks like:
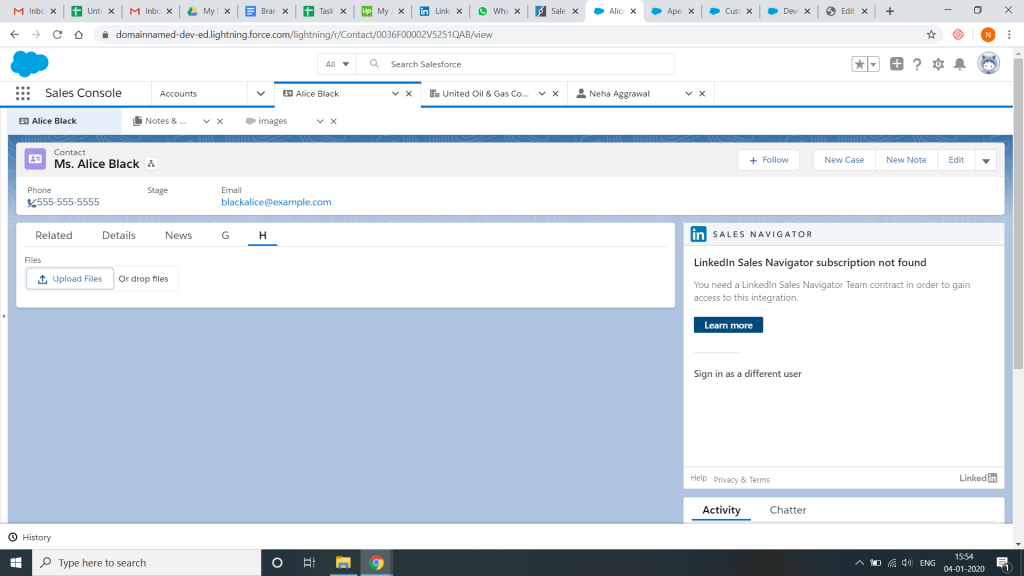
As a first step, create the file input lightning component:
<aura:attribute name="FileList" type="Object"/>
<lightning:input aura:id="file-input" type="file" files="{!v.FileList}" label="Files" name="file" multiple="true" onchange="{! c.handleFilesChange }"/>
We have created an attribute called FileList to get the list of files in our JS controller.
Next, read the files through the following code in the controller and helper:
({
handleFilesChange: function (component, event, helper) {
// This will contain the List of File uploaded data and status
var uploadFile = event.getSource().get("v.files");
var self = this;
var file = uploadFile[0]; // getting the first file, loop for multiple files
var reader = new FileReader();
reader.onload = $A.getCallback(function() {
var dataURL = reader.result;
var base64 = 'base64,';
var dataStart = dataURL.indexOf(base64) + base64.length;
dataURL= dataURL.substring(dataStart);
helper.upload(component, file, dataURL)
});
reader.readAsDataURL(file);
}
});
({
upload: function(component, file, base64Data) {
console.log('helper',file);
var action = component.get('c.uploadFle');
action.setParams({File : base64Data, FileName:file.name, FileSize: file.size, FileType:file.type });
var self = this;
action.setCallback(this, function(actionResult) {
});
$A.enqueueAction(action);
}
})
Helper is sending the base64 data to uploadFile method of the apex controller. The base64 data, file name and file type can be received in String variables and file size in integer.
String can be further changed to Blob data type in the controller or used in other ways. The controller class mentioned here can also be used (with some changes) to upload the file to Google Drive.