In the last post we saw how to create a lightning component to display records of an object in a tabular form. In this post we will use the same example and see how to filter the records based on user input.
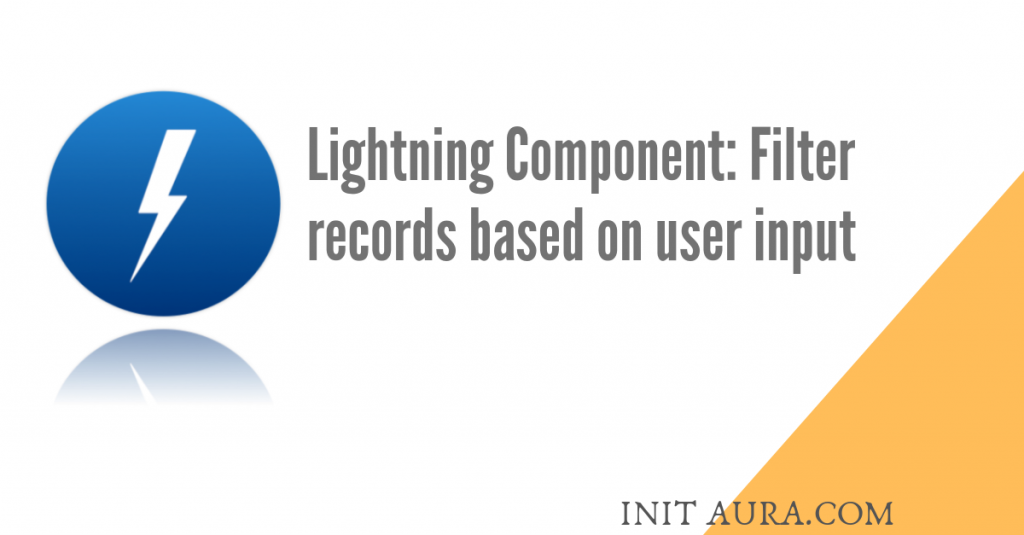
We have our custom object Bank and we have displayed the records in a table. Let’s say I have a custom field called Scale which is a drop down. We will allow the user to choose the value from the drop down, based on which displayed records should be updated.
This is our code for the component:
<aura:component controller="BankController" implements="force:hasRecordId,force:appHostable,flexipage:availableForAllPageTypes" access="global">
<!-- List to store banks data -->
<aura:attribute name="Banks" type="List" />
<!-- Initialization method calls controller to get data -->
<aura:handler name="init" value="{!this}" action="{!c.doInit}" /><div class="slds-col">
<!--tabular data -->
<table class="slds-table slds-table_bordered slds-table_striped slds-table_cell-buffer slds-table_fixed-layout">
<thead>
<tr class="slds-text-heading_label">
<th scope="col">
<div class="slds-truncate" title="Name">Name</div>
</th>
<th scope="col"><div class="slds-truncate" title="Name">Scale</div></th>
</tr>
</thead>
<tbody>
<!-- Use the Apex model and controller to fetch server side data -->
<aura:iteration items="{!v.Banks}" var="bank">
<tr>
<th scope="row">
<div class="slds-truncate" title="{!Bank.Name}">
<!-- Bank name is linked to the Salesforce record page -->
<a href="{!'/lightning/r/Bank__c/'+ Bank.Id + '/view'}" target="_blank">{!Bank.Name}</a></div>
</th>
<td><div class="slds-truncate" title="{!Bank.Scale__c}">{!Bank.Scale__c}</div></td>
<td><div class="slds-truncate" title="{!Bank.Credit_Funding__c}">{!Bank.Credit_Funding__c}</div></td>
</tr>
</aura:iteration>
</tbody>
</table>
</aura:component>
Add the following lines just after the init method:
<div class="slds-col ">
<ui:inputCheckbox aura:id="checkbox1" change="{!c.checkscale}" label="Scale" labelClass="slds-p-horizontal--small slds-float--left"/>
<lightning:combobox aura:id="Scale__c" disabled="true" label="Scale" onchange="{!c.getfilteredbanks}" value="" labelClass="slds-p-horizontal--small slds-float--left"/>
</div>
We have created a checkbox and a combo box. The combo box creates a drop down for the scale field and checkbox enables the combo box on being set to true.
Now for the controller:
({
doInit: function(component, event, helper) {
helper.getBanks(component, event, helper);
}
Add the following lines for the two new functions we have added for checkbox and combo box:
getfilteredbanks:function(component,event,helper){
helper.getfilteredbanks(component,event,helper);
},
checkscale:function(component,event,helper){
helper.checkscale(component,event,helper);
},
This was our helper code which just fetched the bank records from BankController class. We don’t need to add anything to the controller code.
({
// Fetch the banks from the Apex controller and assign the data to banks list declared in our component
getBanks: function(component) {
var action = component.get('c.getBanks');
// Set up the callback
var self = this;
action.setCallback(this, function(actionResult) {
component.set('v.Banks', actionResult.getReturnValue());
});
$A.enqueueAction(action);
}
Add the following lines to the helper class:
//function to enable or disable the drop down based on user selection
checkscale:function(component,event,helper) {
var checkbox1=component.find("checkbox1").get("v.value");
if(checkbox1===true)
component.find("Scale__c").set("v.disabled", false);
if(checkbox1===false){
component.find("Scale__c").set("v.disabled", true);
helper.getfilteredbanks(component,event,helper);
},
// function to get filtered banks based on user input
getfilteredbanks:function(component,event,helper) {
var scale='';
if(component.find("Scale__c").get("v.disabled")==false)
scale=component.find("Scale__c").get("v.value");
var searcharr=[];
if(scale!=='') searcharr["Scale__c"]=scale;
var final_arr=[];
var fullList = component.get("v.Banks");
final_arr=fullList.filter(function(item){
for (var key in searcharr) {
if (item[key] === undefined || item[key] != searcharr[key])
return false;
}
return true;
});
component.set('v.Banks',final_arr);
}
This is how the component looks like. I have created a tab on one of the record pages and added our lightning component. You can check this link to add lightning component to a custom tab on a record page.
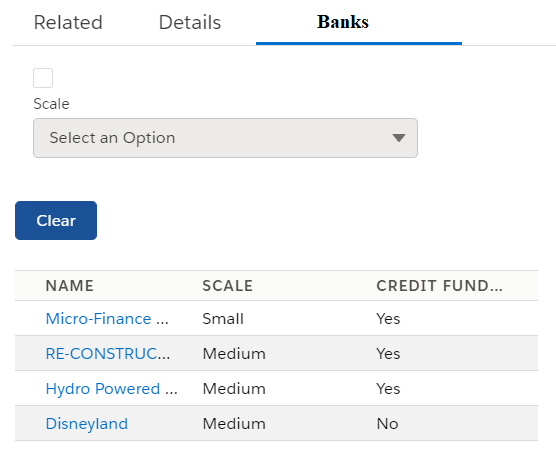
You can filter on more fields as per your need, the fields should be present in the SOQL query in your controller. In the getfilteredbanks function, just add the user input of these fields to searcharr with their keys. And don’t forget to add the fields to the component !!
That’s it, you will not be create lightning component to filter records based on user input.