Auto number field in Salesforce assigns the number sequentially. That means auto number is assigned in series as records are created. For ex: Account records might be assigned Acc-001, Acc-002 and so on.
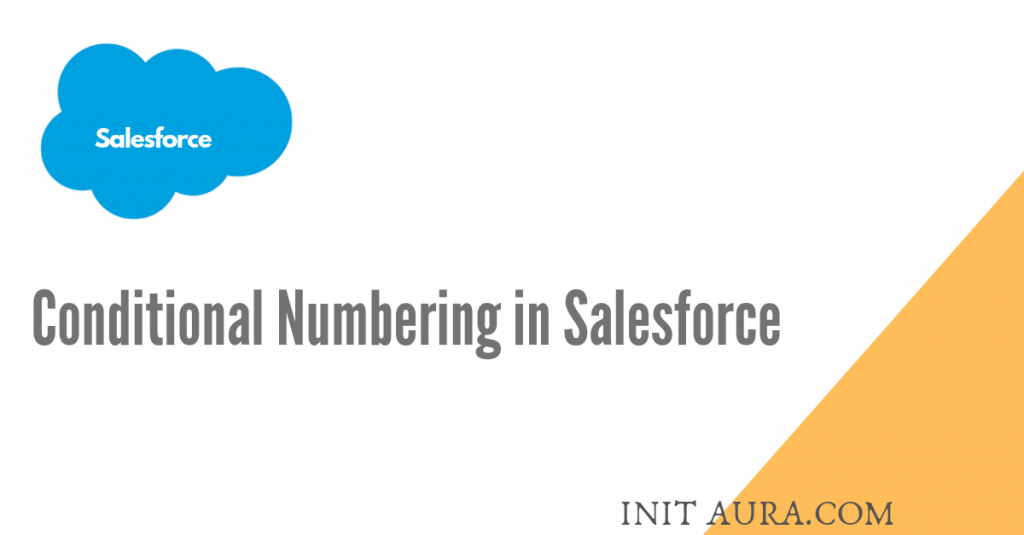
What if we want to use conditional numbering that is separate series based on a field value. For ex: All tasks related to one account should be given sequence T-001, T-002. For tasks of another account, the sequence instead of continuing will start again from 001. So Acc-1 has 2 tasks numbered Acc-1-001 and Acc-1-002. Account Acc-2 has 3 tasks numbered Acc-2-001, Acc-2-002 and Acc-2-003.
I recently had to work on similar requirement. For this I wrote an after insert, before delete trigger on Task.
trigger autoNumber on Task (after insert, before delete) {
UpdateNumber obj=new UpdateNumber();
obj.generateFunc();
}
On insert the trigger checks the Opportunity to see how many tasks exist for the same opportunity. Accordingly it assigns a number to the new task.
On delete, the trigger checks the existing tasks for the Opportunity associated with the deleted task and updates the numbers for all tasks accordingly.
public class UpdateNumber{
public void generateFunc(){
Integer deltasknumber=0;
Integer tasknumber=0;
String a='';
String b='';
Integer count=0; Integer i=0;
Set<Id> TaskSet=new Set<Id>{};
Set<Id> OppSet = new Set<Id>{};
Set<Id> TaskSet1=new Set<Id>{};
Set<Id> OppSet1 = new Set<Id>{};
List<Task> TasktoUpdate= new List<Task>{};
List<Task> TasktoUpdate1= new List<Task>{};
if(trigger.isInsert)
{
Task[] newtsk=Trigger.new;
for(Task temptsk: newtsk)
{
TaskSet.add(temptsk.Id);
OppSet.add(temptsk.whatId);
}
AggregateResult[] result= [Select count(Id)can, WhatId from Task where WhatId in: OppSet and Id not in: TaskSet group by WhatId ];
Map<Id,Integer> map1= new Map<Id,Integer>();
if(result.size()!=0)
{
for(AggregateResult res: result)
{
map1.put((Id)res.get('WhatId'), (Integer)res.get('can'));
}
}
Task[] tsk=[Select Id,What.Name, Task_Number__c from Task where Id in: TaskSet];
Opportunity[] opp=[Select Id, Name from Opportunity where Id in: OppSet];
if(result.size()!=0)
{
for(Opportunity o:opp)
{
if(map1.get(o.Id)!=null) count=map1.get(o.Id)+1; else count=1;
for(Task t:tsk)
{
if(t.WhatId==o.Id)
{
t.Task_Number__c=o.Name+'-00'+count;
TasktoUpdate.add(t);
count++;
}
}
}
}
else
{
for(Opportunity o:opp)
{
count=1;
for(Task t:tsk)
{
if(t.WhatId==o.Id){
t.Task_Number__c=o.Name+'-00'+count;
TasktoUpdate.add(t);
count++;
}
}
}
}
}
else if(trigger.isDelete)
{
Map<Id,String> MapOppTask= new Map<Id,String>();
Map<Id,String> MapOppTask1= new Map<Id,String>();
Task[] deltsk=Trigger.old;
for(Task temptsk: deltsk)
{
TaskSet1.add(temptsk.Id);
OppSet1.add(temptsk.whatId);
}
Task[] tskdeleted=[Select Id,What.Name, Task_Number__c from Task where Id in: TaskSet1 ALL ROWS];
Task[] AllTask=[Select Id ,What.Name, Task_Number__c from Task where WhatId in: OppSet1 and Id not in:TaskSet1 order by CreatedDate];
Opportunity[] opp1=[Select Id, Name from Opportunity where Id in: OppSet1];
for(Task t:tskdeleted)
{
for(Opportunity o: Opp1)
{
if(o.Id==t.WhatId)
MapOppTask.put(t.Id,o.Name);
}
}
for(Task t:AllTask)
{
for(Opportunity o: Opp1)
{
if(o.Id==t.WhatId)
MapOppTask1.put(t.Id,o.Name);
}
}
if(tskdeleted.size()!=0 && Alltask.size()!=0)
for(Opportunity o:Opp1)
{
for(Task t:tskdeleted)
{
if(MapOppTask.get(t.Id)!=null) a=t.Task_Number__c.replace(MapOppTask.get(t.Id),'');
a=a.replace('-','');a=a.replace(' ','');a=a.replace('00','');
deltasknumber=Integer.valueOf(a);
for(Task t1:AllTask)
{
if(MapOppTask1.get(t1.Id)!=null) b=t1.Task_Number__c.replace(MapOppTask1.get(t1.Id),'');
b=b.replace('-','');b=b.replace(' ','');b=b.replace('00','');
tasknumber=Integer.valueOf(b);
if(t.WhatId==o.Id && t1.WhatId==o.Id)
{
if(deltasknumber < tasknumber)
{
tasknumber=deltasknumber;
t1.Task_Number__c=MapOppTask1.get(t1.Id)+'-00'+tasknumber;
TasktoUpdate1.add(t1);
tasknumber++;
deltasknumber=tasknumber;
}
}
}
}
}
}
update TasktoUpdate;
update TasktoUpdate1;
}
}
Conditional Numbering in Salesforce can be implemented with Apex coding or lightning flows. I just like Apex coding better.